A Pointer is used to access the information from a particular location. A Pointer is basically a variable that stores the address of a different variable and then points to them. A pointer can be of different datatypes, for example:- int, float, char, double, etc.
int * ptr ⇾ pointer of integer type
char *ptr ⇾ pointer of char type
float *ptr ⇾ pointer of float type
double *ptr ⇾ pointer of double type
struct pcm *ptr ⇾ pointer of struct type
Why Pointers ?
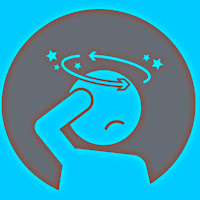
Pointers can more efficiently handle and process the data tables. Also also reduces the length and complexity of a program and also increases the execution speed of a program. Pointers basically used to access the variable in or outside of a function.
Syntax: datatype *pointer_variable_name;
Types of Pointers:
There are 4 types of pointers
- void Pointer:
This is a pointer which has no datatype specified, i.e, it is of void type. We use this pointer when we were not sure about the datatype. By the help of typecasting we can store values of any datatype in this.
LIMITATIONS -
⭆A void pointer cannot directly deferred, so it needs to be typecast.
⭆Pointer Arithmetic cannot be implement on the void pointer.
EXAMPLE -
void *ptr;
int a = 10;
char c = 'A';
ptr = &a;
printf("%d",*(int *)ptr);
ptr= &c;
printf("%c",*(char *)ptr); - Wild Pointer:When a pointer is declared but no value is initialized to the pointer, then this type of pointer is known as Wild pointer. This pointer points the garbage value i.e, it will store the garbage value.
int *arr;
//no value is assigned to arr.
EXAMPLE -
int *ptr;
printf("%d",*ptr);
//here pointer can't find the value and the pointer behaves wildly - NULL Pointer:
An pointer is used to store address but apart from it the pointer can also store "NULL".
EXAMPLE -int *ptr;
ptr = NULL;
Since NULL is not an address, therefore it can't be deferred. - Dangling Pointer:
When a pointer points to an address of that variable whose scope is over then these type of pointers is known as Dangling Pointer.
EXAMPLE -
int *ptr;
{
int a;
ptr = &a;
scanf("%d",ptr);
//here we don't need '&' because pointer is itself an address
printf("%d",*ptr);
//here 'ptr' has the value
}
printf("%d",*ptr);
//here also 'ptr' has the value, just the variable is out of scope
Pointer Arithmetic
In pointers we can use some arithmetic operations:-
- Addition of an integer to a pointer.
- Subtraction of integer to a pointer.
- Subtraction of two pointers.
- Increment and decrement operation on pointers.
- Assignment and Relational operators can be implemented.
NOTE
char a[5];
p=a;
a=p; //it is an error
Since 'a' is a constant pointer, therefore its value cannot be changed.
Some Input/Output for Pointers:
int *arr;
int array[5];
arr = &array;
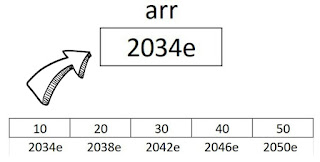
printf("%d",++(*arr));
printf("%d",*(++arr));
printf("%d",++arr);
printf("%d",++(*array));
OUTPUT : 11
20
2034e
11
printf("%d",++array);
// this is an error because ++array means array=array+1;
//because here also value of a constant pointer is changing
printf("%d",*(++array));
// here also ++array means array=array+1;
//because here also value of a constant pointer is changing
Things To Remember
- In Pointers on the time of declaration, we should always use "*".
- Since Pointer is itself an address, therefore '&' operator is not needed.
- Pointer Arithmetic cannot be implemented in void pointer.
- The value of a constant pointer cannot be changed.
To read our post on Function, please click on FUN( ).
To read our post on Array (Part-1) please click on Arr1.
To read our post on Array (Part-1) please click on Arr1.
For giving some suggestion please comment us or check our "Contact us" page. Also more update related to coding will be added in this Blog later on, so please get attached with this Blog. Thank you for your support and time...
0 Comments